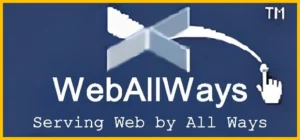
If you’ve spent any time working with a WordPress website, you’ve likely heard the term “related posts.” Related posts are a fantastic way to keep your visitors engaged by showing them more content that aligns with what they’ve already read. Not only does this help reduce bounce rates and increase page views, but it also boosts SEO by improving internal linking.
At WebAllWays, we believe in simplifying SEO strategies. In this guide, we’ll walk you through how to display related posts in WordPress using a combination of plugins and custom code. Whether you’re using a plugin or writing custom code, we’ve got you covered. Additionally, we’ll show you specific code snippets that you can use for GeneratePress users to display random or static related posts.
What Are Related Posts?
Before we dive into how to display related posts, let’s first explore what they are and why they’re important.
Related posts are pieces of content on your website that are shown at the end of an article or within the post itself. They are displayed because they are similar in topic, category, or tags to the content your reader has just viewed. The goal is to provide additional value to your readers by suggesting more content they might find interesting.
- From an SEO perspective, related posts help:
- Improve internal linking, which helps Google crawl and index your content more effectively.
- Increase page views, which signals to search engines that users find your content valuable.
- Enhance user experience, by offering more relevant content based on the reader’s interests.
- Reduce bounce rate, by keeping visitors on your website longer.
Now, let’s get into the “how-to” of displaying related posts.
Why Display Related Posts in WordPress?
Displaying related posts in WordPress is a great way to optimize your site for both users and search engines. Here are some specific reasons why you should add related posts:
Boost User Engagement
When users finish reading a post, they’re more likely to stay on your site if they’re given more content to explore. Related posts offer them exactly that, making it easier to discover more of your work without having to navigate away from the page.
Increase Page Views and Traffic
Related posts often lead to higher page views per session. When users find more relevant content to read, they’re likely to click through to other pages, which leads to more traffic on your website.
Improve SEO and Site Structure
Displaying related posts helps improve your site’s internal linking structure. Internal links are essential for SEO, as they allow search engines to crawl and index your website more effectively.
Enhance the User Experience
When you give readers content that they’re likely to enjoy, you’re improving their experience on your website. A better user experience translates to more loyal visitors and a higher chance of conversions.
Methods for Displaying Related Posts in WordPress
There are several ways to add related posts to your WordPress site. Some methods are user-friendly and require no coding knowledge, while others offer more flexibility for advanced users.
Method 1: Use a WordPress Plugin
The easiest way to display related posts is by using a plugin. This method doesn’t require any coding, and it works for almost any theme. Here are some popular options:
1.1 Related Posts for WordPress Plugin
This plugin automatically shows related posts based on content, categories, or tags. It’s incredibly easy to install and configure. Here’s how to use it:
- Go to your WordPress dashboard.
- Navigate to Plugins > Add New.
- Search for Related Posts for WordPress.
- Install and activate the plugin.
- Go to Settings > Related Posts and configure the plugin’s settings.
1.2 YARPP (Yet Another Related Posts Plugin)
YARPP is another popular plugin that offers advanced algorithms to display related posts. You can choose between showing related posts as thumbnails or in a list format. After installing the plugin, go to the plugin’s settings and configure it to display related posts according to your needs.
1.3 Jetpack by WordPress.com
Jetpack offers many features, including the ability to display related posts automatically at the end of articles. To enable related posts:
- Install and activate Jetpack.
- Go to Jetpack > Settings.
- Under the Traffic tab, enable Related Posts.
If you’re using GeneratePress, Jetpack’s related posts feature integrates seamlessly with the theme, making it easy to set up without additional customization.
Method 2: Manually Add Related Posts Using Custom Code
For more control and customization, you can manually add related posts by editing your theme’s functions.php file. This method allows you to tailor the related posts display to suit your exact needs.
Here are two useful code snippets for GeneratePress users. These codes allow you to display related posts based on categories and give you flexibility in how you present them.
Displaying Random Related Posts (Changes on Refresh)
If you want to display related posts randomly each time the page is refreshed, use the following code:
// Add related posts function to functions.php function display_related_posts() { // Get the current post's categories $categories = get_the_category(); if ($categories) { // Get the category IDs $category_ids = array(); foreach ($categories as $category) { $category_ids[] = $category->term_id; } // Query for random related posts $args = array( 'category__in' => $category_ids, // Posts from the same category 'posts_per_page' => 3, // Number of related posts to show 'post__not_in' => array(get_the_ID()), // Exclude the current post 'orderby' => 'rand', // Order by random (random posts each time) 'post_type' => 'post', // Only posts ); $related_posts = new WP_Query($args); // If related posts are found if ($related_posts->have_posts()) { echo '<div class="related-posts">'; echo '<h3>Related Posts</h3>'; echo '<ul>'; while ($related_posts->have_posts()) { $related_posts->the_post(); // Get post excerpt $excerpt = get_the_excerpt(); echo '<li>'; // Only wrap the title in the link echo '<a href="<?php echo get_permalink(); ?>">'; echo '<h4><?php echo get_the_title(); ?></h4>'; echo '</a>'; // Close the link here, so only the title is clickable. // Display the post excerpt (short content) if ($excerpt) { echo '<p><?php echo $excerpt; ?></p>'; } echo '</li>'; } echo '</ul>'; echo '</div>'; wp_reset_postdata(); } } } // Hook the related posts function to display after the post content add_action('generate_after_main_content', 'display_related_posts', 10);
This code randomly selects related posts from the same category as the current post and displays them. Each time the page is refreshed, the related posts will change.
Displaying Static Related Posts (Doesn’t Change on Refresh)
If you’d rather display static related posts (i.e., posts that don’t change on refresh), use the following code:
// Add related posts function to functions.php function display_related_posts() { // Get the current post's categories $categories = get_the_category(); if ($categories) { // Get the category IDs $category_ids = array(); foreach ($categories as $category) { $category_ids[] = $category->term_id; } // Query for related posts $args = array( 'category__in' => $category_ids, // Posts from the same category 'posts_per_page' => 3, // Number of related posts to show 'post__not_in' => array(get_the_ID()), // Exclude the current post 'orderby' => 'date', // Order by date (most recent) 'order' => 'DESC', // Descending order (newest first) 'post_type' => 'post', // Only posts ); $related_posts = new WP_Query($args); // If related posts are found if ($related_posts->have_posts()) { echo '<div class="related-posts">'; echo '<h3>Related Posts</h3>'; echo '<ul>'; while ($related_posts->have_posts()) { $related_posts->the_post(); // Get post excerpt $excerpt = get_the_excerpt(); echo '<li>'; // Only wrap the title in the link echo '<a href="<?php echo get_permalink(); ?>">'; echo '<h4><?php echo get_the_title(); ?></h4>'; echo '</a>'; // Close the link here, so only the title is clickable. // Display the post excerpt (short content) if ($excerpt) { echo '<p><?php echo $excerpt; ?></p>'; } echo '</li>'; } echo '</ul>'; echo '</div>'; wp_reset_postdata(); } } } // Hook the related posts function to display after the post content add_action('generate_after_main_content', 'display_related_posts', 10);
Customizing the Hook for Your Theme
If you’re not using the GeneratePress theme, you can easily adjust the hook to fit your theme. The add_action()
function in the code hooks into specific actions within WordPress themes. For example, generate_after_main_content
is specific to GeneratePress. If you’re using a different theme, you’ll need to find the appropriate hook that corresponds to your theme’s structure.
Here’s an example of how you can modify the hook:
- For a theme that uses
wp_footer
:
- For a theme that uses
add_action('wp_footer', 'display_related_posts', 10);
- For a theme with custom hooks: Check your theme’s documentation or files (like
single.php
orcontent-single.php
) to find the correct hook.
FAQ: Displaying Related Posts in WordPress
1. How do related posts benefit SEO?
Related posts improve your internal linking structure, which makes it easier for search engines to crawl and index your content. By linking to other pages within your site, you help Google understand the context and relevance of your articles.
2. Can I customize the related posts display?
Yes! You can change the number of related posts displayed, modify how they’re sorted (random or by date), and customize their appearance. If you’re using custom code, you can tailor it to match your site’s design.
3. Will related posts slow down my website?
If you’re using a plugin, it’s important to monitor your site’s performance. Some plugins can slow down page load times, especially if they add extra queries. Custom coding your related posts can be faster, but you should always test your site’s speed.
4. Do I need to use a plugin to display related posts?
No, you can achieve this by writing custom code in your theme’s functions.php file. However, plugins are a great option for users who want a quick and easy setup without any coding.
5. Can I display related posts for other post types besides blog posts?
Yes! The code provided here targets posts, but you can modify the post_type
parameter to include custom post types, like pages or products, by adjusting the query arguments in the code.
Conclusion
Displaying related posts in WordPress is an excellent strategy for improving user engagement, boosting SEO, and reducing bounce rates. Whether you prefer using a plugin or custom code, related posts can be a game-changer for your site.
For GeneratePress users, the provided code snippets are a perfect way to customize your related posts section. And remember, you can easily modify the hooks to suit other themes!
Have fun experimenting with related posts, and feel free to reach out to WebAllWays if you need help with WordPress development or SEO!