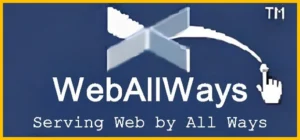
Understanding the Basics of WordPress Forms
Before diving into the technical implementation, it’s important to understand the fundamental components of a contact form and how WordPress manages forms:
- HTML – Defines the structure and layout of the form fields.
- PHP – Processes the form data on the server side, handles validation, and sends emails.
- CSS – Styles the form to match your WordPress website design.
By combining these elements, you can create a contact form that is both functional and visually appealing.
Preparing Your WordPress Environment
1. Backing Up Your Website
Always back up your website before making changes. This precaution ensures that you can restore your site if any issues arise. Use a reliable backup plugin or service to create a complete backup of your site.
2. Creating a Child Theme
Using a child theme prevents changes from being overwritten when updating your parent theme. Here’s the exact way how to create a child theme:
Step 1: Create a Child Theme Directory
Go to /wp-content/themes/
and create a new folder named your-theme-child
.
Step 2: Create a Stylesheet
In the your-theme-child
folder, create a file named style.css
with the following content:
/*
Theme Name: Your Theme Child
Template: your-theme
*/
Replace your-theme
with your website’s parent theme.
Step 3: Create a Functions File
In the same folder, create a file named functions.php
and add the following code to enqueue the parent theme’s stylesheet:
<?php
function your_theme_child_enqueue_styles() {
wp_enqueue_style('parent-style', get_template_directory_uri() . '/style.css');
}
add_action('wp_enqueue_scripts', 'your_theme_child_enqueue_styles');
?>
3. Creating a Custom Page Template
Create a new file named page-contact.php
in your child theme folder to serve as your contact form template:
<?php
/**
* Template Name: Contact Form
*/
get_header(); ?>
<main id="main" class="site-main">
<div class="contact-form">
<?php
// Display the contact form
?>
</div>
</main>
<?php get_footer(); ?>
Creating a Contact Form Using PHP and HTML
Step 1: Creating a New Page Template
Define your page template by opening page-contact.php
and setting up the basic structure. This file will render the contact form.
Step 2: Adding HTML Code for the Form
Add the HTML structure for your contact form within the contact-form
div:
<form id="contact-form" action="<?php echo esc_url($_SERVER['REQUEST_URI']); ?>" method="post">
<label for="cf-name">Name:</label>
<input type="text" id="cf-name" name="cf-name" required>
<label for="cf-email">Email:</label>
<input type="email" id="cf-email" name="cf-email" required>
<label for="cf-message">Message:</label>
<textarea id="cf-message" name="cf-message" rows="5" required></textarea>
<input type="submit" name="cf-submit" value="Send Message">
<div id="form-response"></div>
</form>
Step 3: Handling Form Submissions with PHP
Add PHP code to process form submissions. This includes sanitizing inputs, sending the email, and providing feedback:
<?php
if (isset($_POST['cf-submit'])) {
$name = sanitize_text_field($_POST['cf-name']);
$email = sanitize_email($_POST['cf-email']);
$message = esc_textarea($_POST['cf-message']);
$to = 'your-email@example.com'; // Replace with your email address
$subject = 'Contact Form Submission';
$headers = array('Content-Type: text/html; charset=UTF-8');
$body = "Name: $name<br>Email: $email<br>Message: $message";
if (wp_mail($to, $subject, $body, $headers)) {
echo '<p class="form-success">Thank you for your message. We will get back to you soon.</p>';
} else {
echo '<p class="form-error">There was an error sending your message. Please try again later.</p>';
}
}
?>
Step 4: Adding CSS for Styling
To style your contact form, add CSS to the style.css
file in your child theme:
.contact-form form {
max-width: 600px;
margin: 0 auto;
padding: 1em;
border: 1px solid #ddd;
border-radius: 5px;
}
.contact-form label {
display: block;
margin-bottom: 0.5em;
}
.contact-form input,
.contact-form textarea {
width: 100%;
padding: 0.5em;
margin-bottom: 1em;
}
.contact-form input[type="submit"] {
background-color: #0073aa;
color: white;
border: none;
cursor: pointer;
}
.contact-form input[type="submit"]:hover {
background-color: #005177;
}
.form-success {
color: green;
}
.form-error {
color: red;
}
Implementing AJAX for Form Submission
To enhance user experience by preventing page refreshes upon form submission, you can implement AJAX. This allows the form to submit data and display success messages dynamically.
Step 1: Adding AJAX Script
Include an AJAX script in your theme’s functions.php
to handle the form submission. Add the following code to enqueue the script:
function enqueue_ajax_scripts() {
wp_enqueue_script('ajax-script', get_stylesheet_directory_uri() . '/js/ajax-form.js', array('jquery'), null, true);
wp_localize_script('ajax-script', 'ajax_object', array('ajax_url' => admin_url('admin-ajax.php')));
}
add_action('wp_enqueue_scripts', 'enqueue_ajax_scripts');
Create a JavaScript file named ajax-form.js
in a js
folder within your child theme directory:
jQuery(document).ready(function($) {
$('#contact-form').on('submit', function(e) {
e.preventDefault();
var formData = $(this).serialize();
$.ajax({
type: 'POST',
url: ajax_object.ajax_url,
data: formData + '&action=process_contact_form',
success: function(response) {
$('#form-response').html(response);
$('#contact-form')[0].reset();
},
error: function() {
$('#form-response').html('<p class="form-error">There was an error processing your request. Please try again later.</p>');
}
});
});
});
Step 2: Updating PHP for AJAX Handling
Modify your theme’s functions.php
to handle the AJAX request:
function process_contact_form() {
$name = sanitize_text_field($_POST['cf-name']);
$email = sanitize_email($_POST['cf-email']);
$message = esc_textarea($_POST['cf-message']);
$to = 'your-email@example.com'; // Replace with your email address
$subject = 'Contact Form Submission';
$headers = array('Content-Type: text/html; charset=UTF-8');
$body = "Name: $name<br>Email: $email<br>Message: $message";
if (wp_mail($to, $subject, $body, $headers)) {
echo '<p class="form-success">Thank you for your message. We will get back to you soon.</p>';
} else {
echo '<p class="form-error">There was an error sending your message. Please try again later.</p>';
}
wp_die();
}
add_action('wp_ajax_process_contact_form', 'process_contact_form');
add_action('wp_ajax_nopriv_process_contact_form', 'process_contact_form');
Validating and Securing the Form
1. Form Validation
Ensure that your form validates input data both client-side and server-side:
- Client-Side Validation: Use HTML5 attributes like
required
andtype
to enforce basic validation. - Server-Side Validation: Implement additional checks in your PHP code to verify email formats and sanitize inputs.
2. Security Measures
Nonces: Use WordPress nonces to protect against CSRF attacks. Add a nonce field to your form:
<?php wp_nonce_field('contact_form_action', 'contact_form_nonce'); ?>
Verify the nonce in your PHP processing code:
if (!isset($_POST['contact_form_nonce']) || !wp_verify_nonce($_POST['contact_form_nonce'], 'contact_form_action')) {
die('Security check failed');
}
Sanitization and Escaping: Always sanitize and escape user inputs to prevent malicious code execution. Use functions like sanitize_text_field()
, sanitize_email()
, and esc_textarea()
.
Testing the Contact Form
Thorough testing ensures that your contact form operates as expected:
- Functionality Test: Verify that the form processes data correctly and sends emails.
- Email Delivery Test: Check if emails are received at the designated address and contain the correct information.
- Error Handling Test: Test how the form handles invalid inputs and error scenarios.
Troubleshooting Common Issues
Let’s know the common issues and how to troubleshoot them:
- Email Not Received: Check your spam folder and ensure that the email server is configured correctly. Verify that
wp_mail()
is functioning properly. - Form Not Displaying: Ensure the custom page template is correctly applied and that there are no syntax errors in your PHP or HTML code.
- Validation Errors: Confirm that all form fields have the appropriate attributes and that server-side validation is working correctly.
Conclusion
Creating a contact form in WordPress without a plugin provides a streamlined and customizable solution that can enhance your site’s performance and security. By following the steps outlined in this guide, you can develop a fully functional contact form with advanced features like AJAX-based form submission.
This approach not only gives you greater control over the form’s functionality and appearance but also ensures that your site remains lightweight and efficient. Proper validation and security measures will help protect your form from potential threats and ensure a smooth user experience.
FAQs
- Q1: Can I use a contact form template from another site and modify it for my WordPress site?
- A1: Yes, you can adapt a contact form template from another site, but make sure to customize the code to fit within the WordPress environment. Test thoroughly and apply appropriate validation and security measures.
- Q2: What are the benefits of creating a contact form without a plugin?
- A2: Creating a contact form without a plugin reduces security vulnerabilities, decreases site load time, and provides more control over the form’s functionality and design.
- Q3: How can I integrate CAPTCHA into my custom contact form?
- A3: To integrate CAPTCHA, use services like Google reCAPTCHA. Add the CAPTCHA code to your form and validate the CAPTCHA response in your PHP code.
- Q4: Can I add additional fields to the contact form?
- A4: Yes, you can add extra fields by modifying the HTML form code. Ensure you update the PHP processing code to handle these new fields appropriately.
- Q5: Is it necessary to use a child theme for creating a contact form?
- A5: While not strictly necessary, using a child theme is recommended to prevent overwriting changes when updating the parent theme. It provides a safe environment for customization.